Tuesday, December 12, 2023
Friday, August 19, 2022
Street Runner 2D in Unity
Download Sprites for Asset Store
Car_Spawner Script
Coin_Spawner Script
Game_Controller Script
Player_Movement Script
Road_Movement Script
Scene_Controller Script
Score_Manager Script
Share_Score Script
Watch Video
Thursday, July 21, 2022
Wednesday, April 13, 2022
Chrome Dinosaur Game Hack
Saturday, September 25, 2021
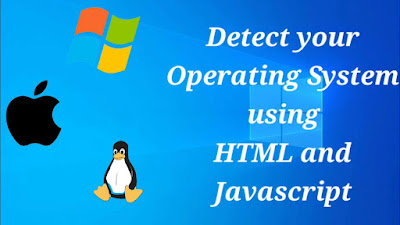
Javascript Code:
Create a new javascript file and name it OSDetector.js. Then write the following codes:
HTML Code:
Create a new html file and name it index.html. Then write the following codes:
Then in visual studio code, install "Live Server" extension.
Then come to index.html and right click on the screen and select "Open with live server" option.
Then a new blank tab will be opened. There, right click on the screen and click on "Inspect" option.
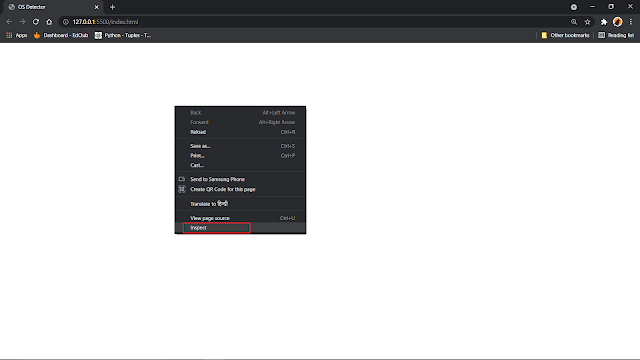
After that you will get a console option at top. Click on it.
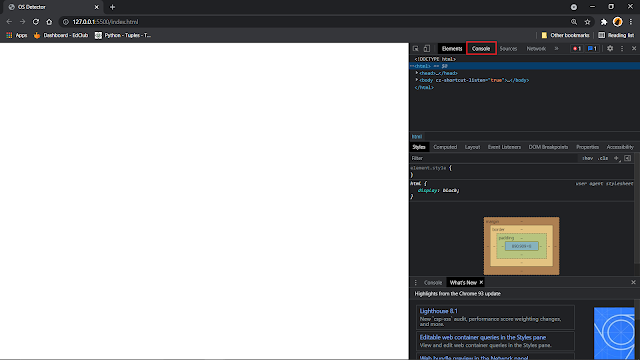
There you will get your operating system name.
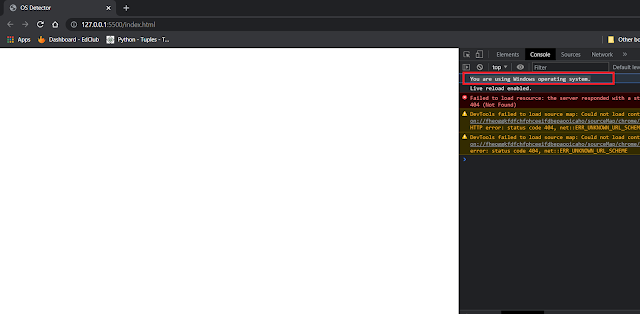
Thursday, September 9, 2021
Step I: Open this link in any browser: https://code.visualstudio.com/
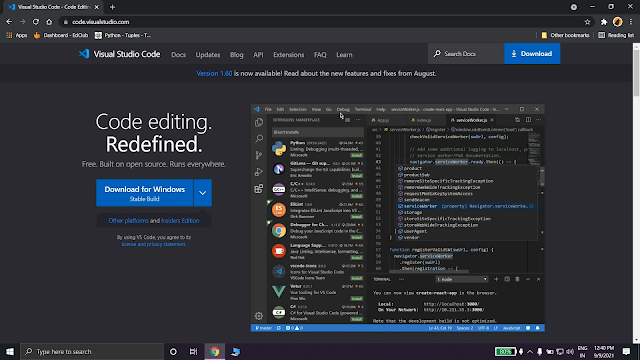
Step II: You will get "Download for Windows" button. You can also download visual studio code by clicking on that for 64 bits else click on that arrow beside the button.
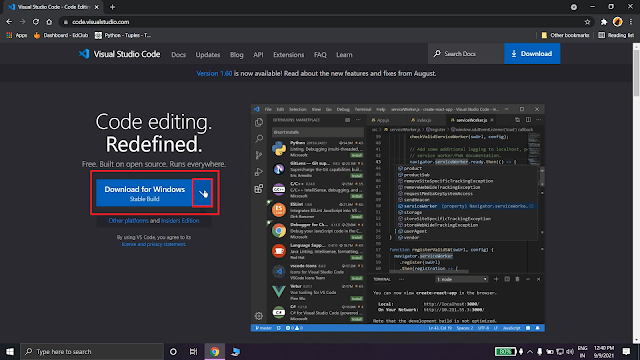
Step III: Now, after that, you can download visual studio code for macOS, windows x64 and linux x64 by clicking the download buttons on the right side. You can download stable version or insider version as your choice. I prefer stable version to download.
Else, you can click on 'other downloads' at the bottom to download for 32 bit or for ARM.
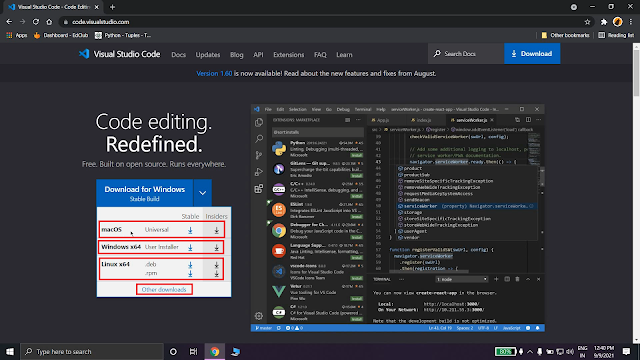
Step IV: After clicking on 'other downloads', you will get an interface like given below. Now you can download visual studio code for windows, linux or mac. You will get many options there so you can select them according to your choice and download it.
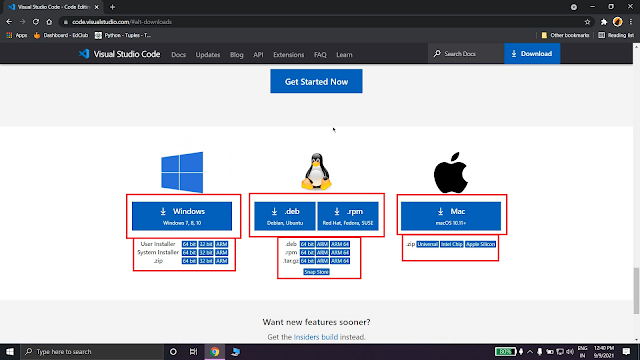
Step V: After clicking any of it, the download will start automatically within 15 seconds. If download didn't start then click on 'direct download link' to download it again.
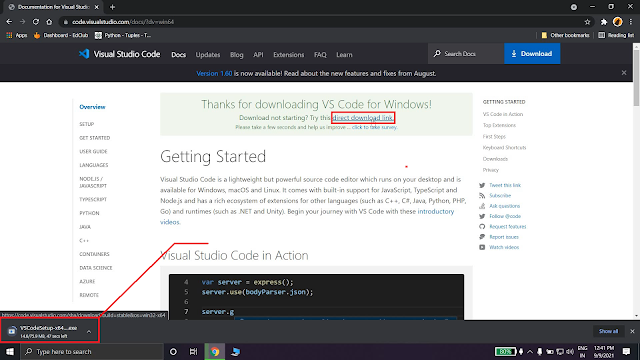
Step VI: After downloading it, open it and accept the License Agreement and click Next.
Step VI: Select the path where your visual studio code will install. I prefer let it be set default. Then click Next.
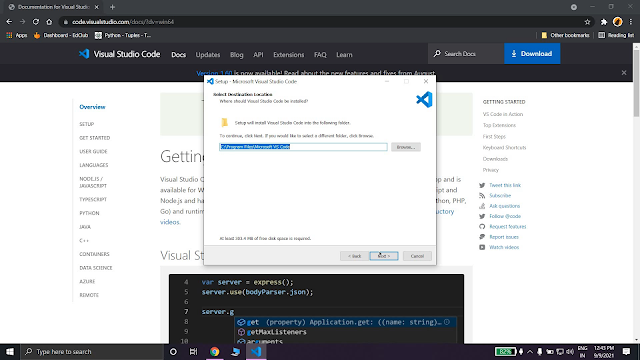
Step VII: If you want to create a folder of Visual Studio code in Start Menu then click Next else check that check box at bottom and then click Next.
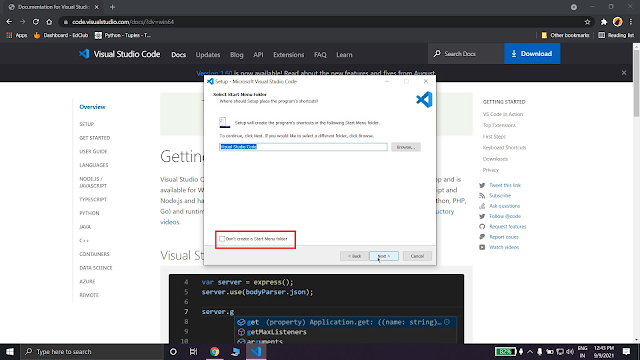
Step VIII: If you want to create a shortcut of visual studio code in desktop then check the first box else uncheck it. then check all the rest check box and click next.
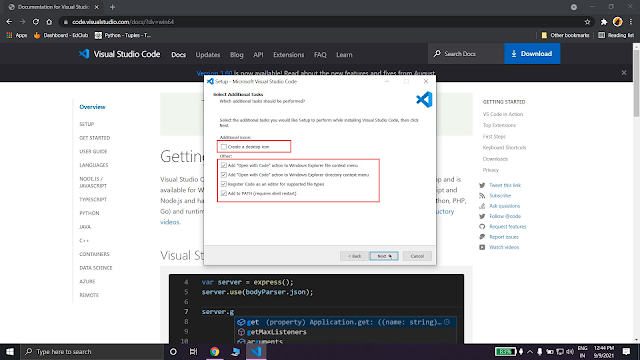
Step IX: Verify your settings and click on install button.
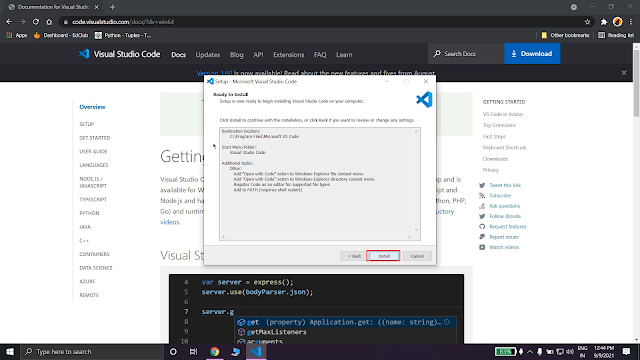
Step X: At last after installation completes, click on Finish. Your visual studio is now installed successfully. Now, enjoy it.
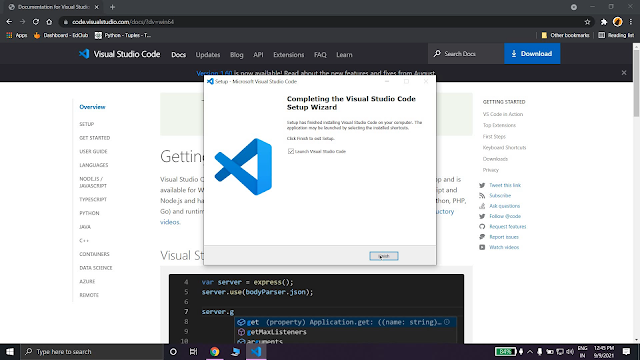
Video:
Sunday, August 22, 2021
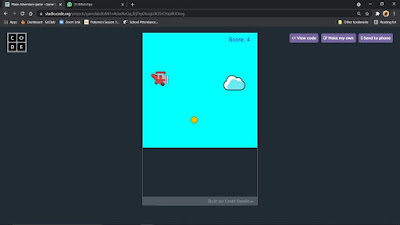
Codes:
var plane = createSprite(50,200);
plane.setAnimation('<Animation Name>');
plane.scale = 0.7;
plane.visible = false;
var coinGroup = createGroup();
var cloudGroup = createGroup();
var gameOver = createSprite(200,150);
gameOver.setAnimation('<Animation Name>');
gameOver.visible = false;
var getReady = createSprite(200,200);
getReady.setAnimation("<Animation Name>");
getReady.visible = false;
var gameState = 'start';
var score = 0;
var hiScore = 0;
function Movement(){
plane.velocityX = 0;
plane.velocityY = 0;
if(keyDown("up")){
plane.velocityY = -10
}
if(keyDown("down")){
plane.velocityY = 10
}
if(keyDown("RIGHT_ARROW")){
plane.velocityX = 10
}
if(keyDown("LEFT_ARROW")){
plane.velocityX = -10
}
}
function SpawnCoins(){
if(World.frameCount%80 === 0){
var rand = random(10,390)
var coin = createSprite(410,rand);
coin.setAnimation("<Animation Name>");
coin.velocityX = -5;
coin.scale = 0.4;
coin.lifetime = 100;
coinGroup.add(coin)
}
}
function SpawnClouds(){
if(World.frameCount%110 === 0){
var rand = random(10,390)
var cloud = createSprite(410,rand);
cloud.setAnimation("<Animation Name>");
cloud.velocityX = -5;
cloud.scale = 0.2;
cloud.lifetime = 100;
cloudGroup.add(cloud);
}
}
function Score(){
if(plane.isTouching(coinGroup)){
score++;
coinGroup.destroyEach();
}
}
function HiScore(){
if(score>hiScore){
hiScore = score
}
}
function GameOver(){
if(plane.isTouching(cloudGroup)){
coinGroup.destroyEach();
cloudGroup.destroyEach();
gameState = 'over'
}
}
function draw() {
background('cyan');
drawSprites();
createEdgeSprites();
plane.collide(edges);
if(gameState === 'start'){
getReady.visible = true;
gameOver.visible = false;
plane.visible = false;
if(World.frameCount%50 === 0){
gameState = 'play'
}
}
if(gameState === 'play'){
getReady.visible = false;
plane.visible = true;
Movement();
SpawnCoins();
SpawnClouds();
Score();
HiScore();
GameOver();
fill("blue");
textSize(20)
text('Score: '+score,300,30)
}
if(gameState === 'over'){
plane.visible = false;
gameOver.visible = true;
fill("blue");
textSize(30)
text('High Score: '+hiScore,100,250)
text('Score: '+score,140,300)
text('Press ENTER to restart',50,350)
if(keyDown('enter')){
gameState = 'start';
plane.x = 50;
plane.y = 200;
score = 0;
}
}
}